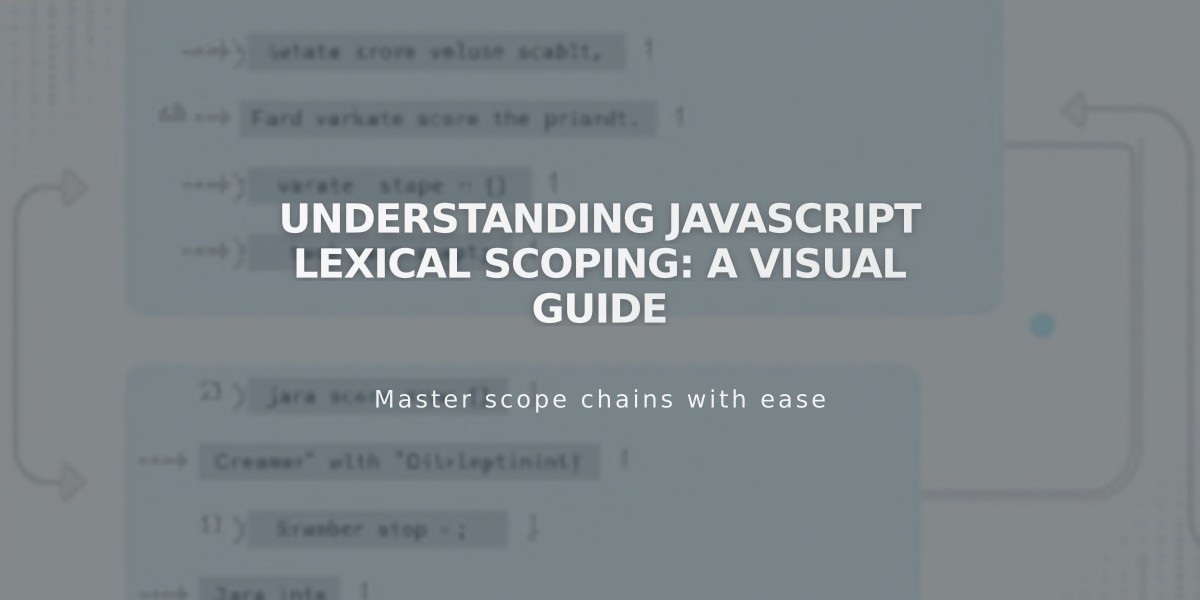
Understanding JavaScript Lexical Scoping: A Visual Guide
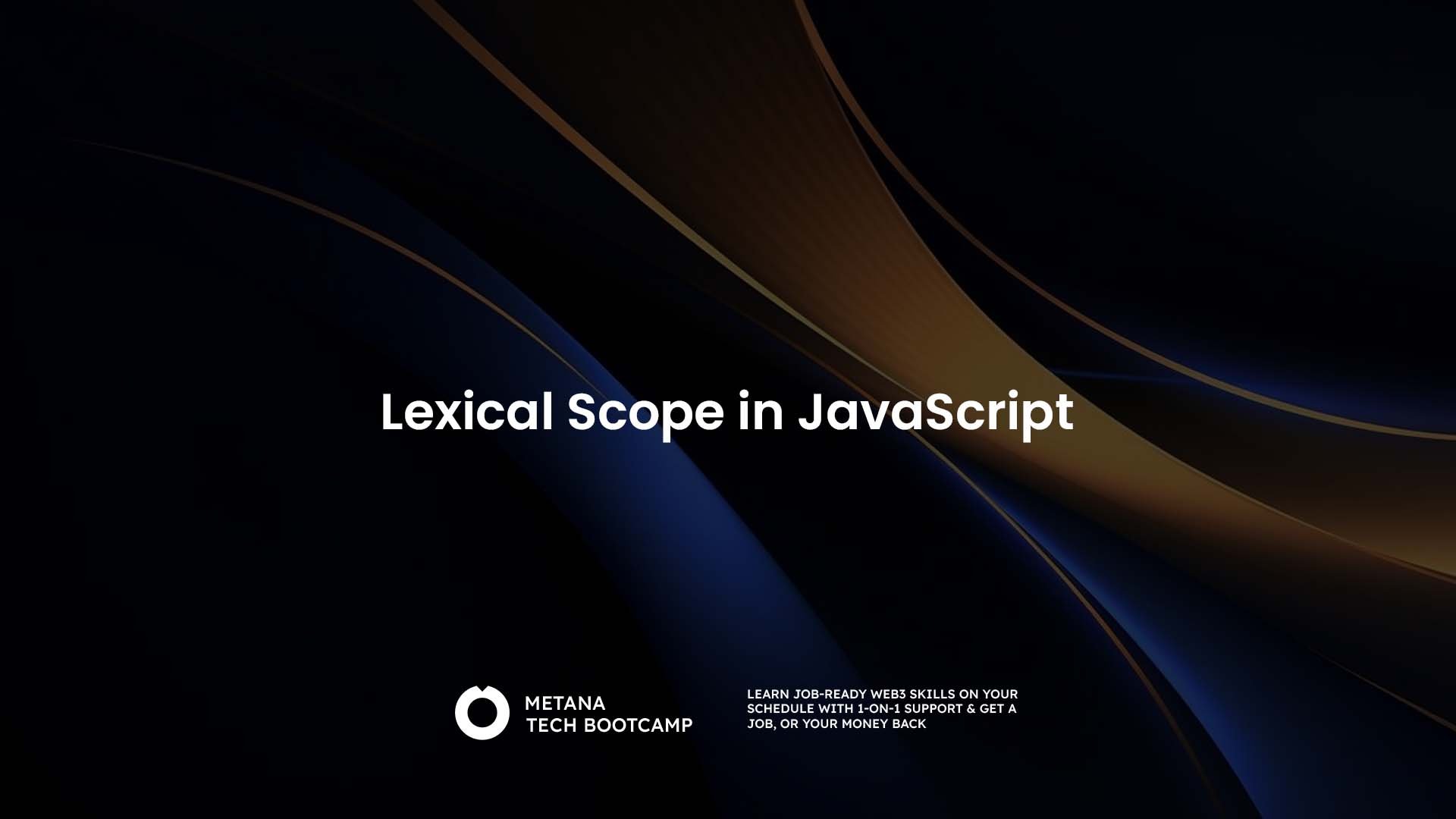
JavaScript Lexical Scope diagram
Lexical scope, also known as static scope, determines how variable names are resolved in nested functions. In JavaScript, when you declare a variable, it's accessible within its immediate function or block, as well as any nested functions.
Variables are resolved by looking first within the innermost scope, then progressively moving outward to find the first matching declaration. This process continues until reaching the global scope.
Here's how lexical scope works:
const globalVariable = "I'm global"; function outer() { const outerVariable = "I'm in outer"; function inner() { const innerVariable = "I'm in inner"; console.log(innerVariable); // Accessible console.log(outerVariable); // Accessible console.log(globalVariable); // Accessible } inner(); }
Key points about lexical scope:
- Inner functions have access to variables in their outer scope
- Outer functions cannot access variables in inner scopes
- Variables with the same name in inner scopes take precedence over outer scopes
- The scope is determined at the time of function definition, not execution
Understanding lexical scope is crucial for:
- Avoiding naming conflicts
- Managing variable accessibility
- Writing more maintainable code
- Implementing closures effectively
- Preventing unintended variable leaks
This scoping mechanism makes JavaScript's closure pattern possible, allowing for data privacy and state management in applications.
Related Articles
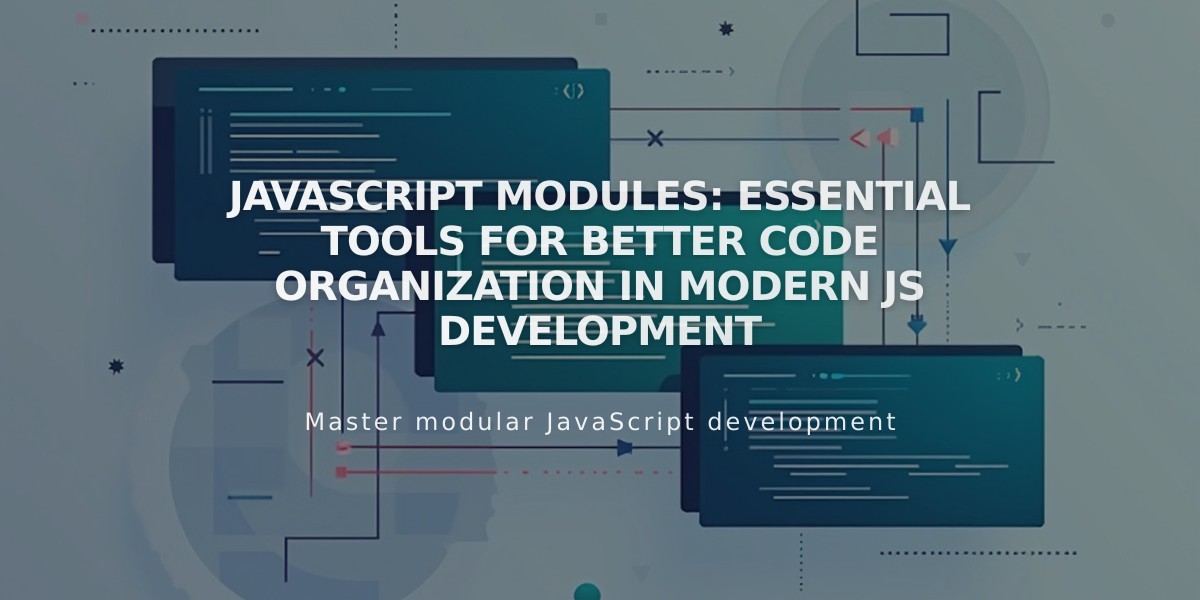
JavaScript Modules: Essential Tools for Better Code Organization in Modern JS Development
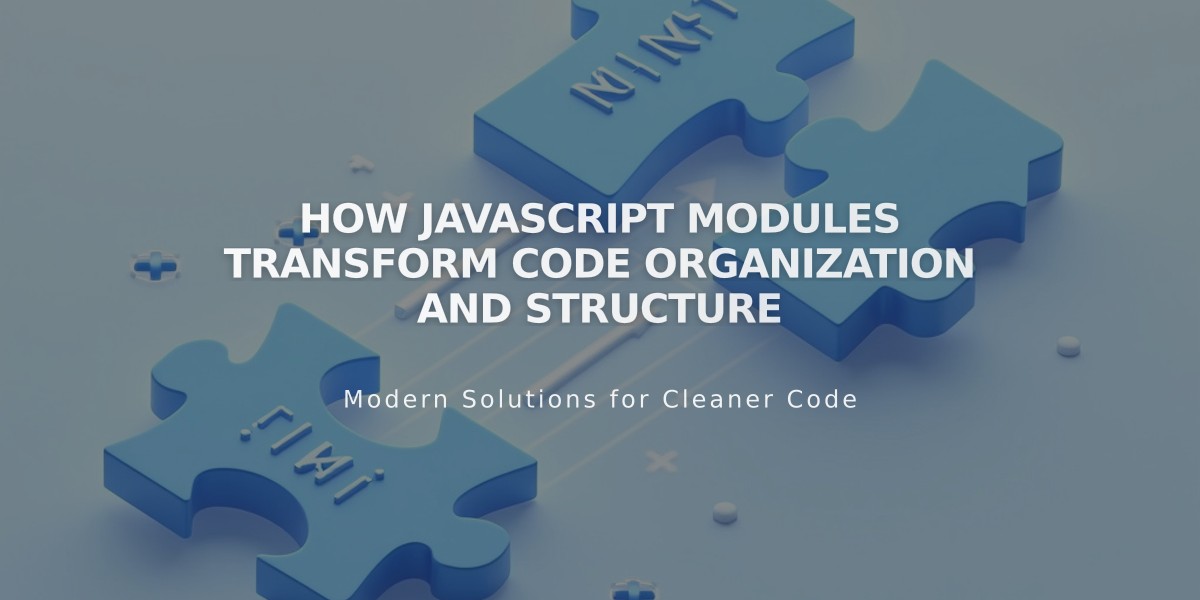