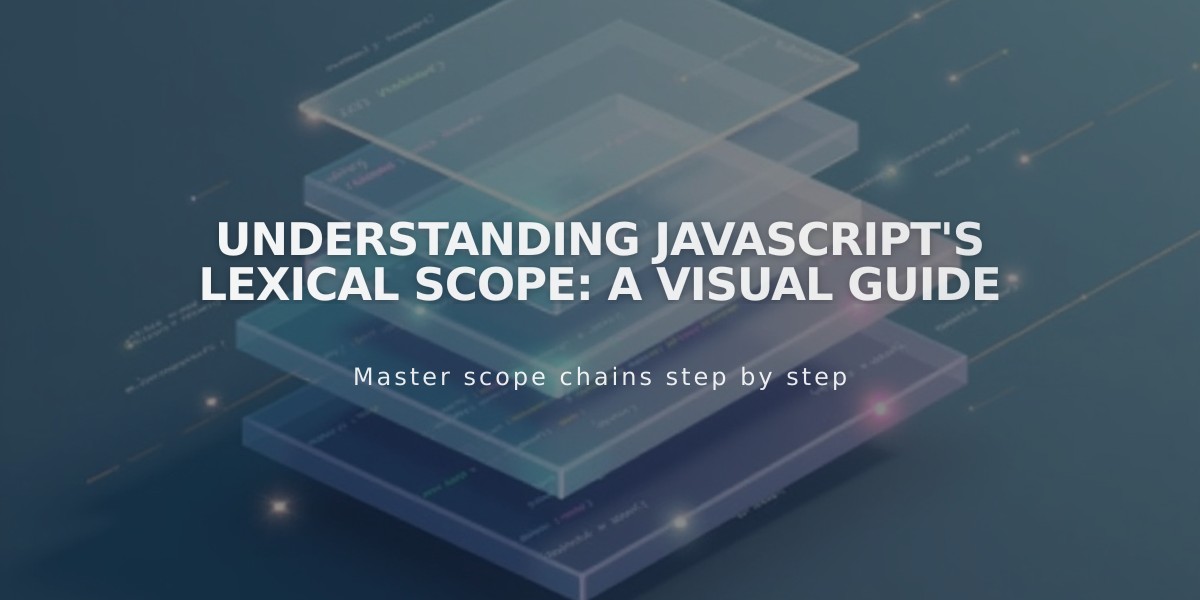
Understanding JavaScript's Lexical Scope: A Visual Guide
JavaScript's lexical scope defines how variable names are resolved in nested functions. Variables declared in outer functions are accessible to functions defined within them, creating a scope chain that determines variable accessibility.
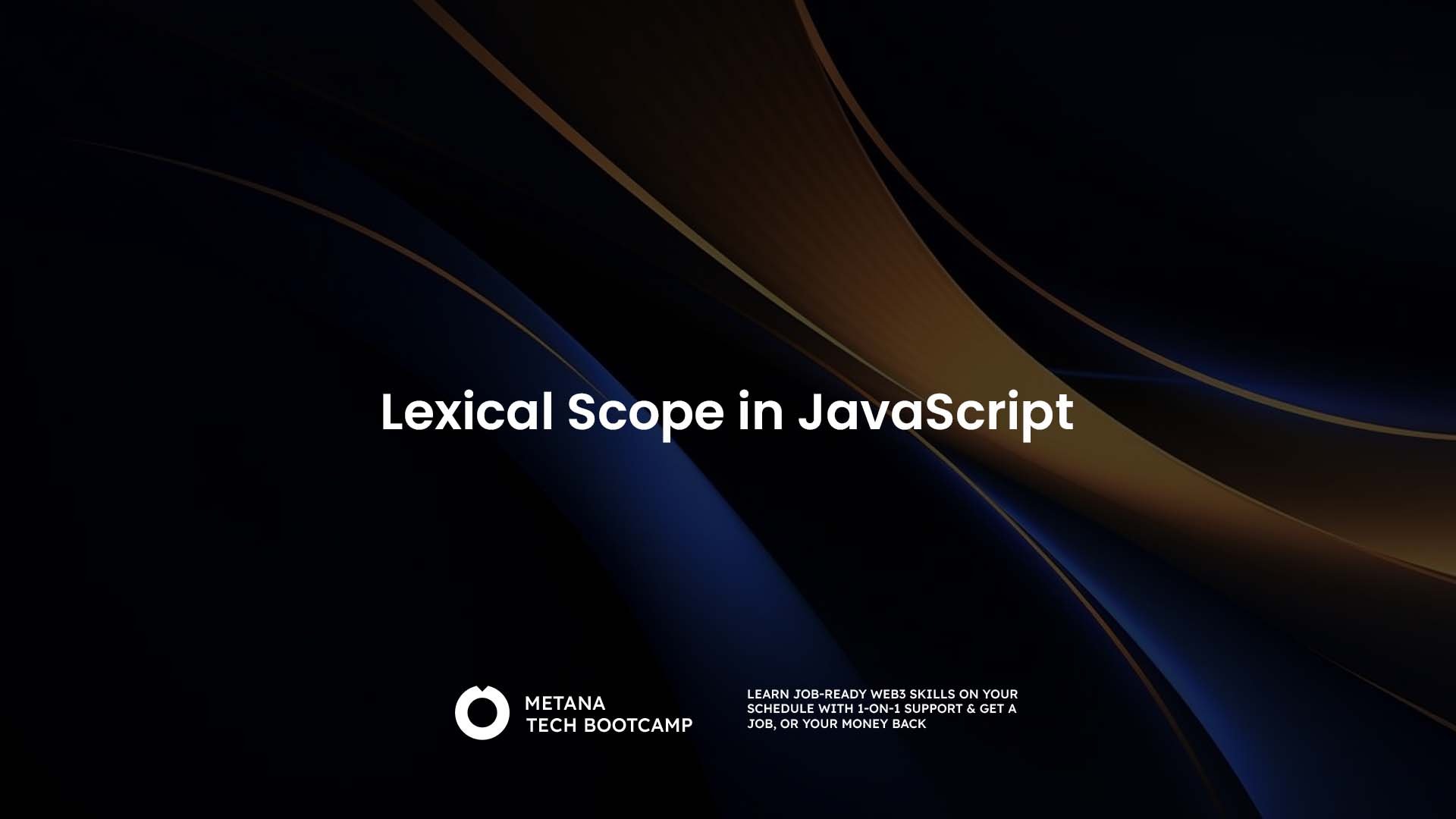
JavaScript Lexical Scope diagram
The scope chain follows these key principles:
- Inner functions can access variables from their outer scope
- Outer functions cannot access variables from inner scopes
- Variables with the same name take precedence in their local scope
- Global variables have the lowest precedence
Here's how lexical scoping works in practice:
const globalVar = "I'm global"; function outerFunction() { const outerVar = "I'm from outer"; function innerFunction() { const innerVar = "I'm from inner"; console.log(innerVar); // Accessible console.log(outerVar); // Accessible console.log(globalVar); // Accessible } innerFunction(); console.log(innerVar); // Not accessible }
Understanding lexical scope is crucial for:
- Avoiding naming conflicts
- Managing variable accessibility
- Writing maintainable code
- Implementing closures effectively
- Preventing unintended variable leaks
Best practices include:
- Using block scope with let and const
- Avoiding global variables when possible
- Keeping functions focused and small
- Understanding the scope chain hierarchy
- Leveraging closures intentionally
This scoping mechanism makes JavaScript code more predictable and helps prevent unintended variable access across different parts of an application.
Related Articles
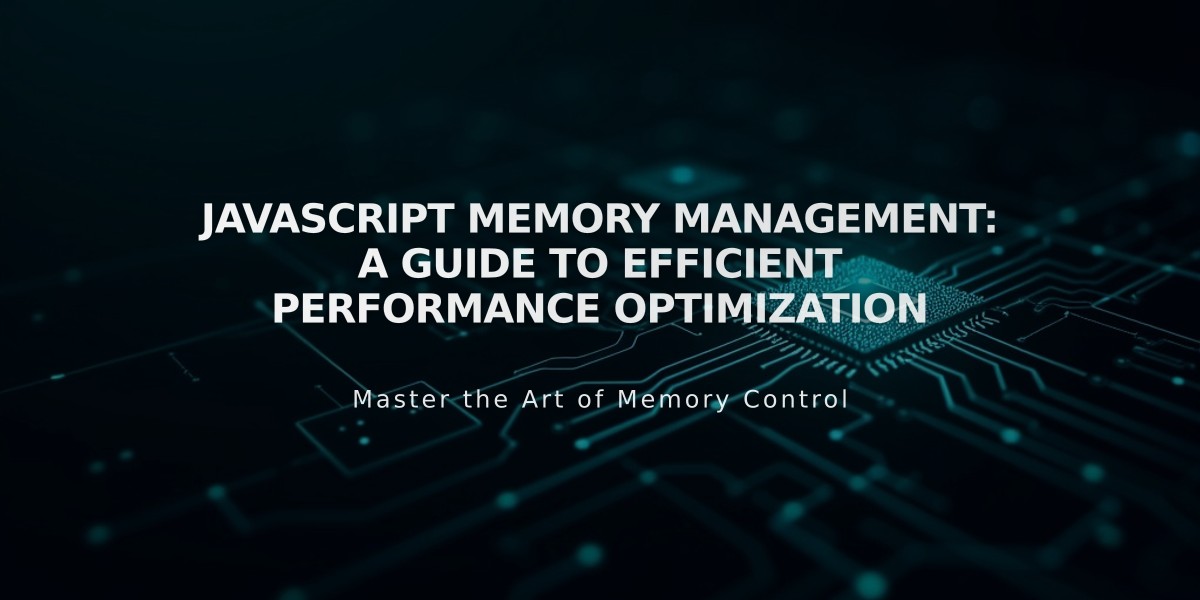
JavaScript Memory Management: A Guide to Efficient Performance Optimization
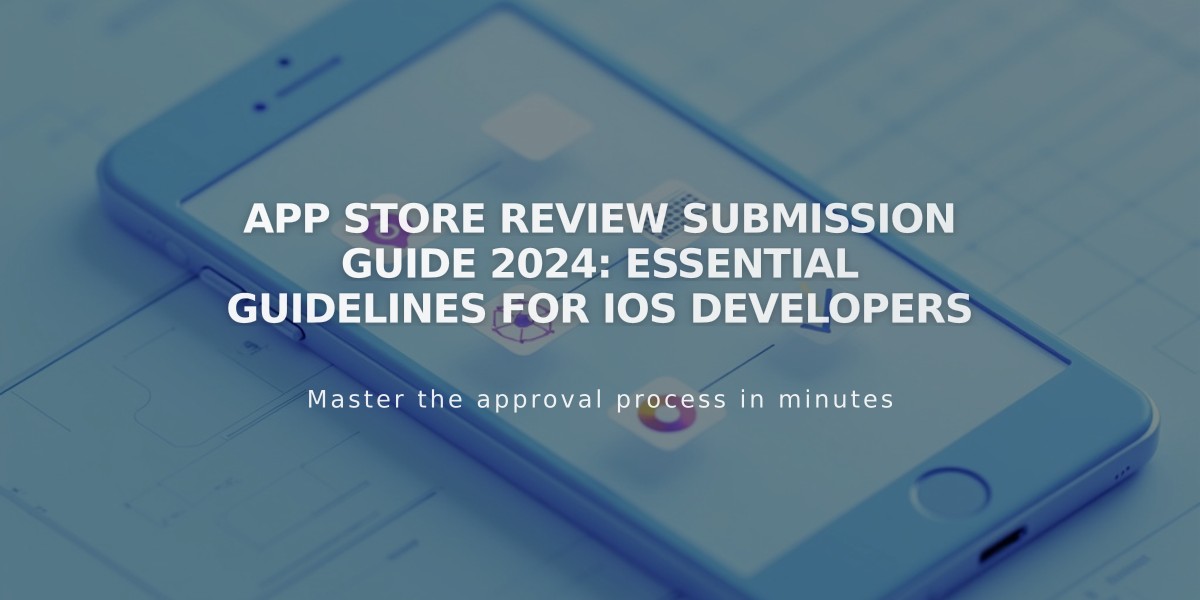