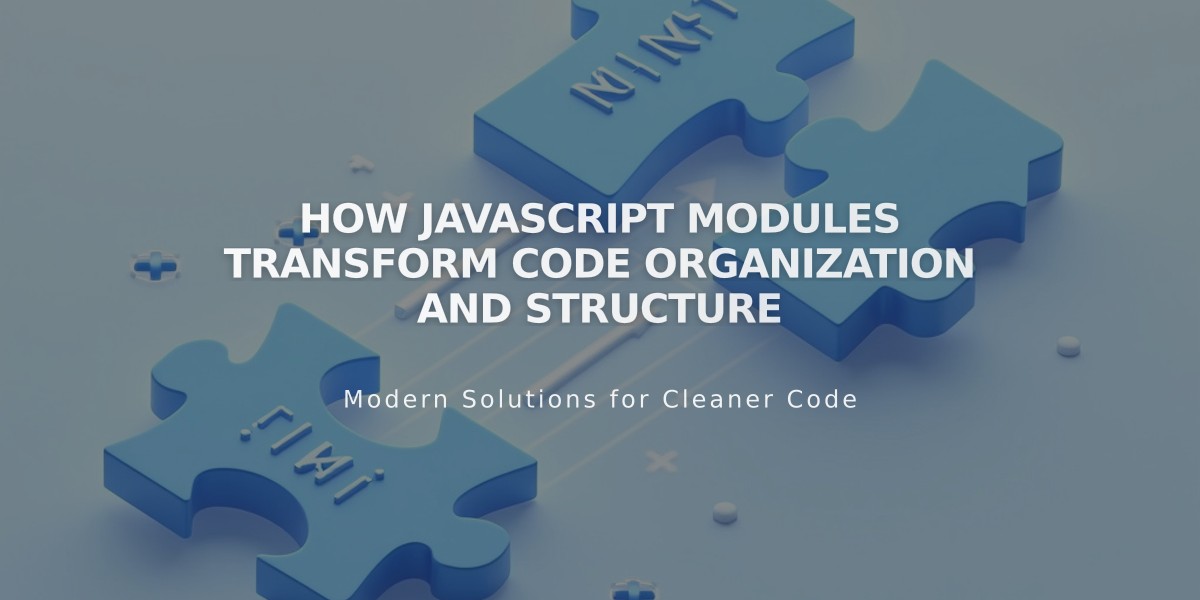
How JavaScript Modules Transform Code Organization and Structure
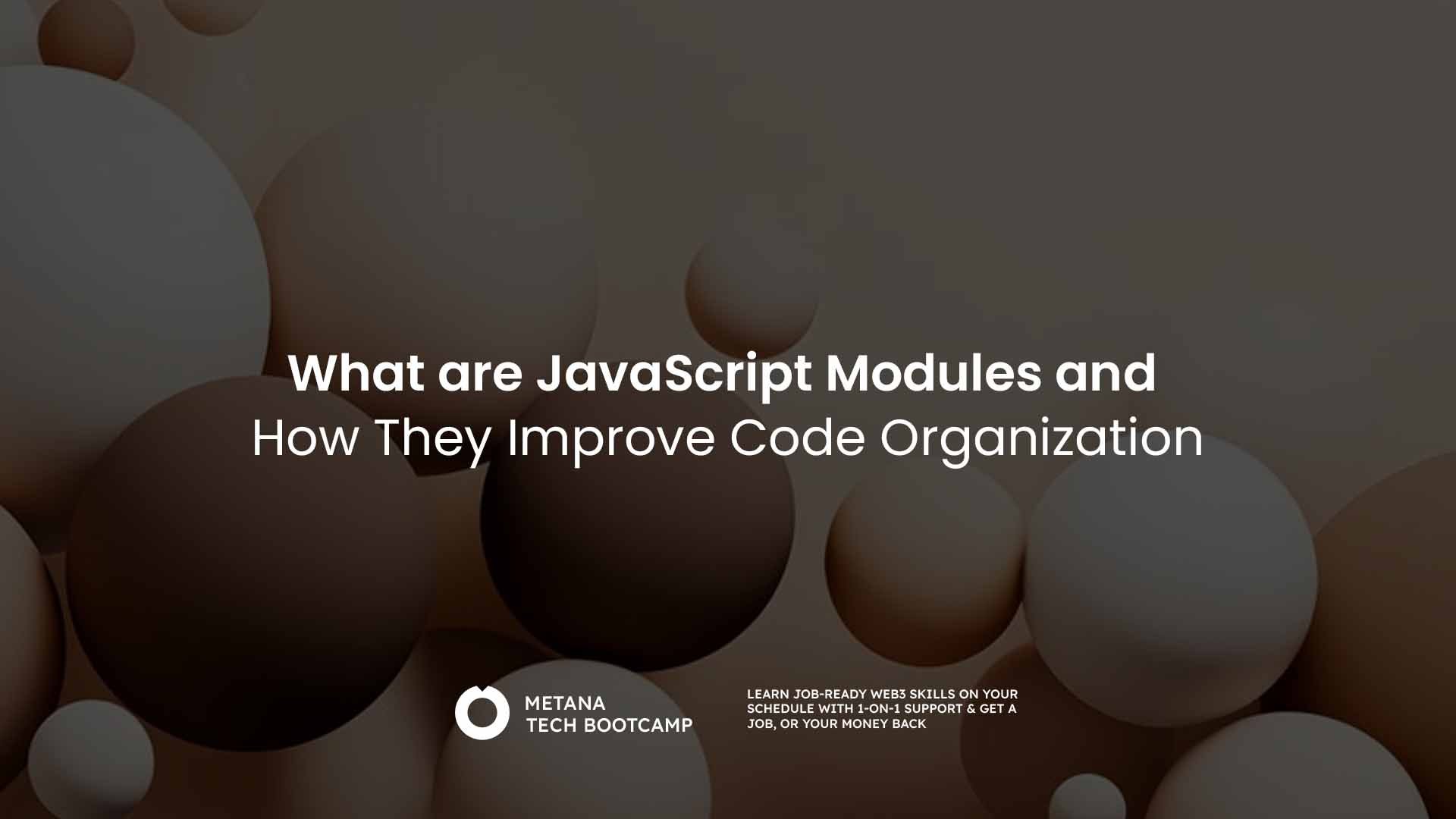
JavaScript modules code structure diagram
JavaScript modules are self-contained units of code that encapsulate related functionality, variables, and classes. They provide a structured way to organize code by separating it into manageable, reusable components.
Modules help solve common problems in JavaScript development:
- Avoiding naming conflicts through scope isolation
- Managing dependencies effectively
- Improving code maintainability
- Enabling code reuse across projects
- Simplifying testing and debugging
Using modules involves two key concepts:
- Export: Making code available for use in other files
- Import: Accessing code from other modules
Here's how to use modules:
// math.js export const add = (a, b) => a + b; export const subtract = (a, b) => a - b; // main.js import { add, subtract } from './math.js'; console.log(add(5, 3)); // Output: 8
Benefits of using modules:
- Better Organization: Each module focuses on a specific functionality
- Encapsulation: Private variables and functions stay hidden
- Dependency Management: Clear imports show what each file needs
- Code Reusability: Share functionality across different parts of your application
- Performance: Modern bundlers optimize module loading
Best practices for module usage:
- Keep modules focused on a single responsibility
- Use clear, descriptive names for exports
- Minimize dependencies between modules
- Document module interfaces
- Export only what's necessary
Modern JavaScript frameworks like React, Vue, and Angular heavily rely on modules for component-based architecture, making them essential for contemporary web development.
Understanding and effectively using JavaScript modules is crucial for writing maintainable, scalable applications in today's development landscape.
Related Articles
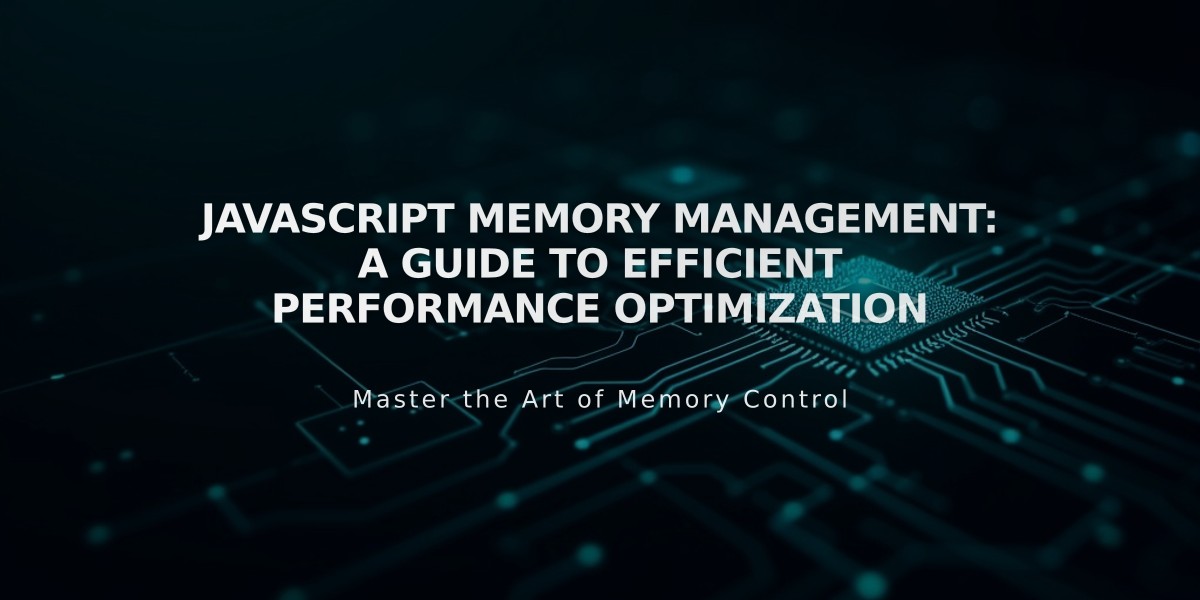
JavaScript Memory Management: A Guide to Efficient Performance Optimization
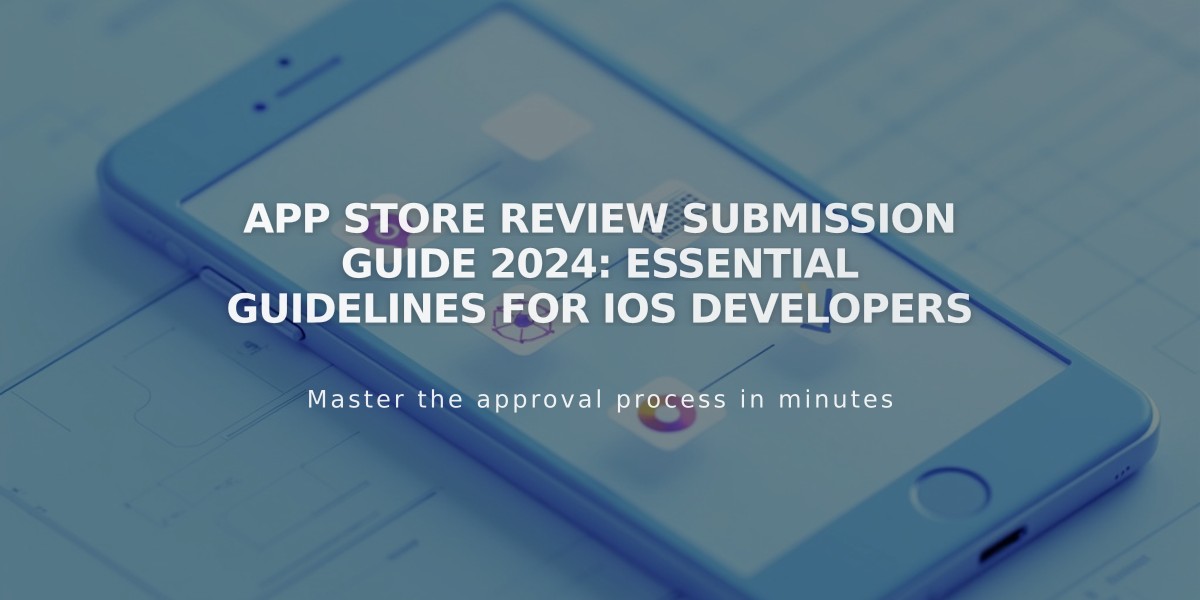