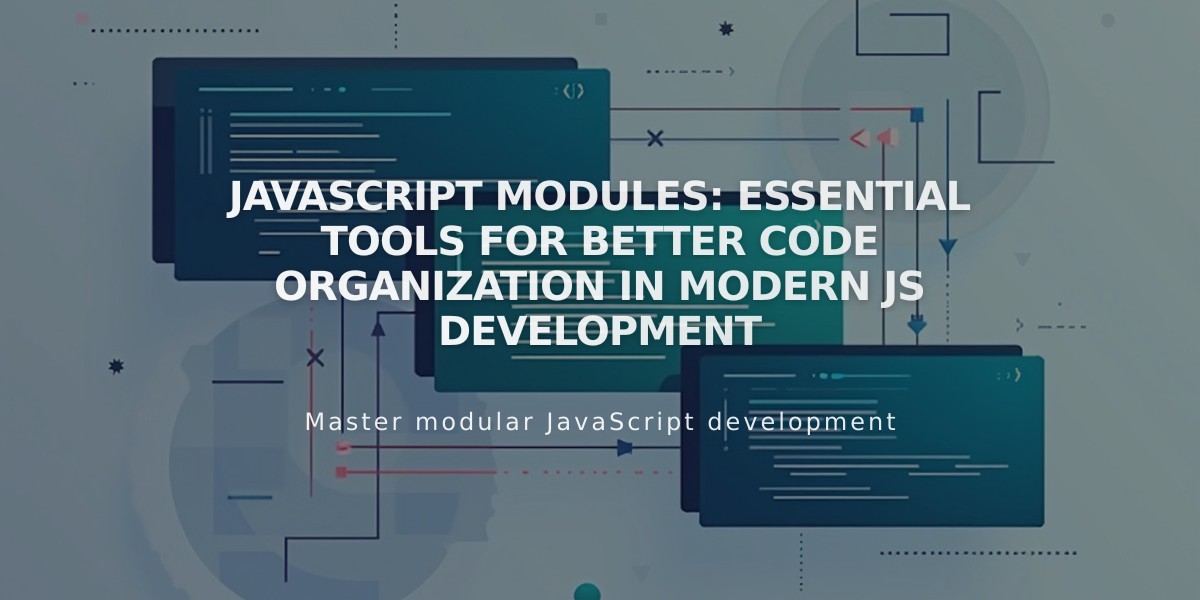
JavaScript Modules: Essential Tools for Better Code Organization in Modern JS Development
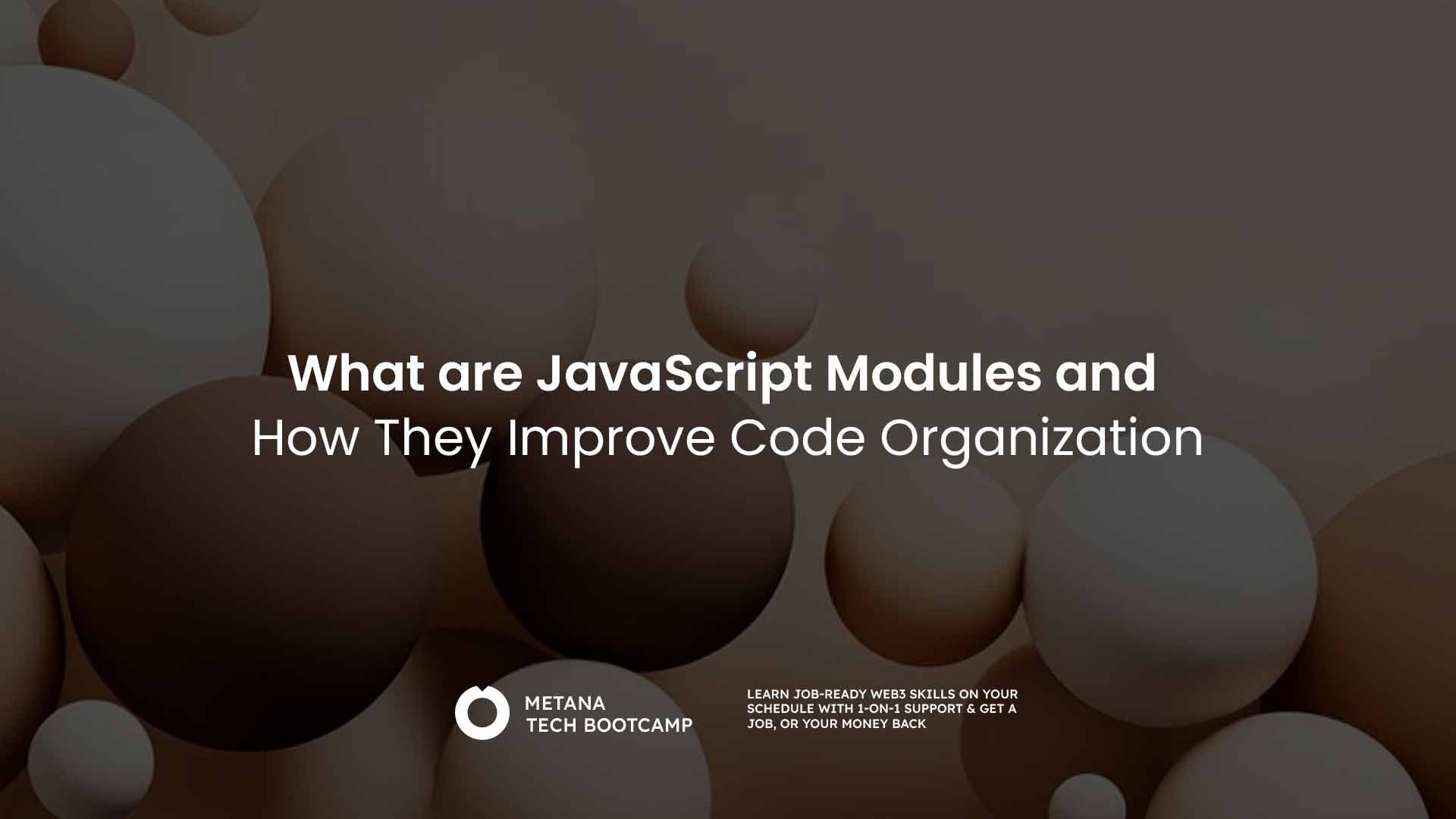
JavaScript modules code structure diagram
JavaScript modules are self-contained units of code that encapsulate related functions, variables, and classes. They allow developers to split code into manageable, reusable pieces that can be easily maintained and shared across different parts of an application.
Modules bring several key benefits to JavaScript development:
- Encapsulation: Keep code private and expose only what's necessary through exports
- Dependency Management: Clearly define relationships between different parts of your code
- Namespace Protection: Avoid naming conflicts by keeping variables scoped to their modules
- Code Organization: Structure code logically and maintain cleaner project architecture
Using modules in your JavaScript project is straightforward:
// math.js export const add = (a, b) => a + b; export const subtract = (a, b) => a - b; // main.js import { add, subtract } from './math.js';
To implement modules in your project:
- Add
type="module"
to your script tag - Use
export
to make items available to other modules - Use
import
to access exported items from other modules - Ensure your server supports ES modules
Modern browsers support modules natively, providing better performance through:
- Code splitting
- Tree shaking
- Deferred loading
- Better caching
Best practices for working with modules:
- Export a single responsibility per module
- Keep module dependencies simple and clear
- Use descriptive file names that reflect module contents
- Maintain a flat module structure when possible
- Document module interfaces and dependencies
Modules have transformed JavaScript development by enabling better code organization, maintenance, and scalability. They're now a fundamental part of modern JavaScript applications and essential for building robust, maintainable code bases.
Related Articles
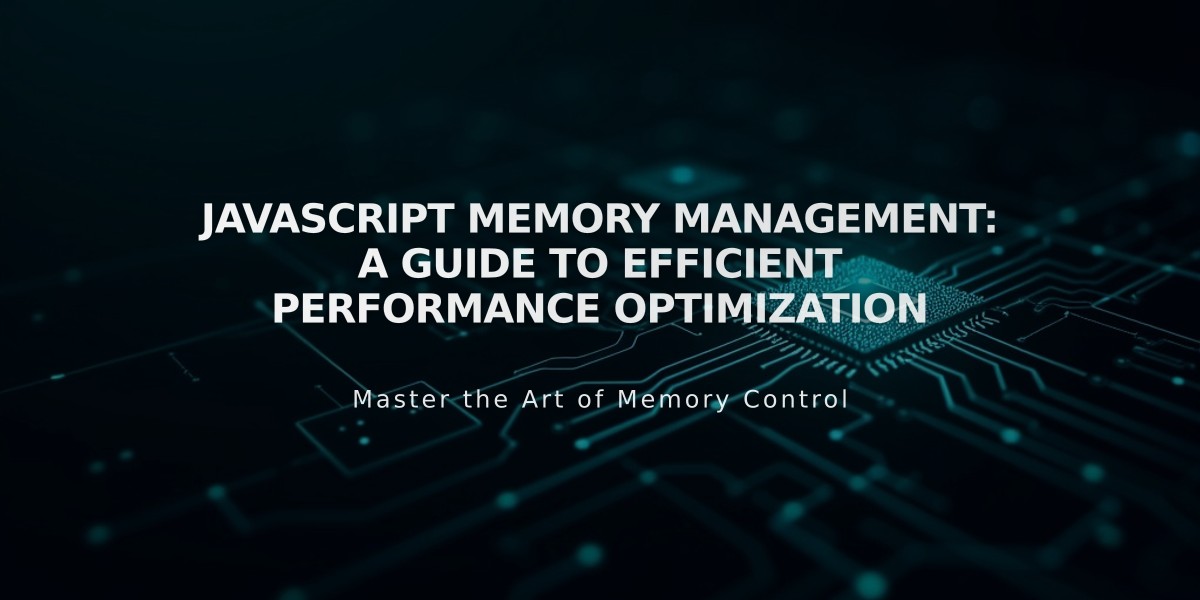
JavaScript Memory Management: A Guide to Efficient Performance Optimization
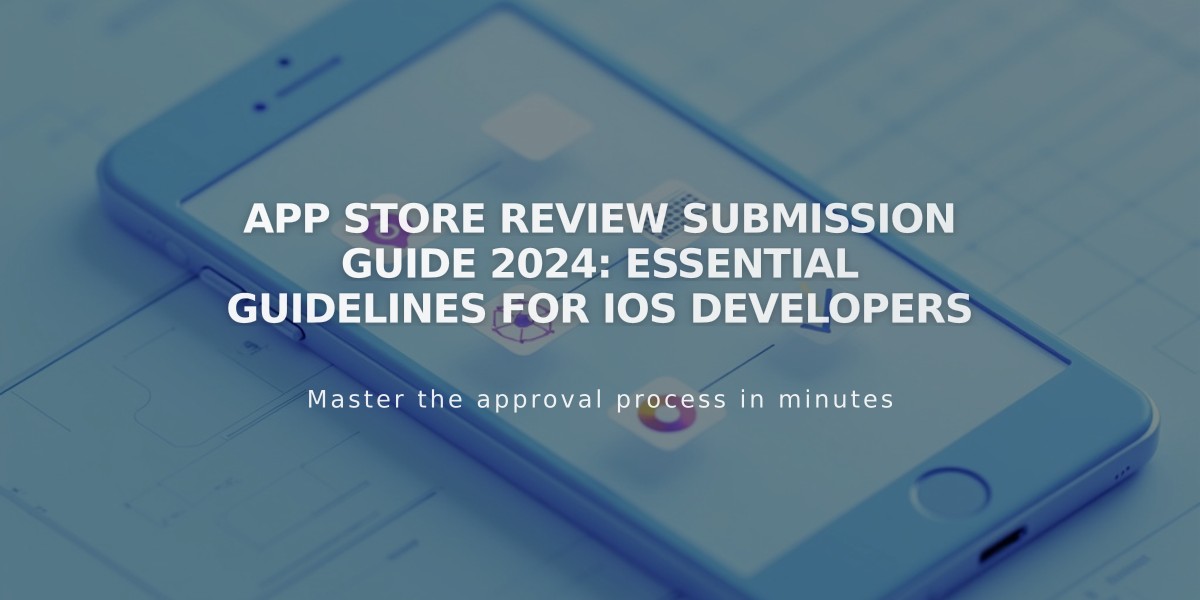