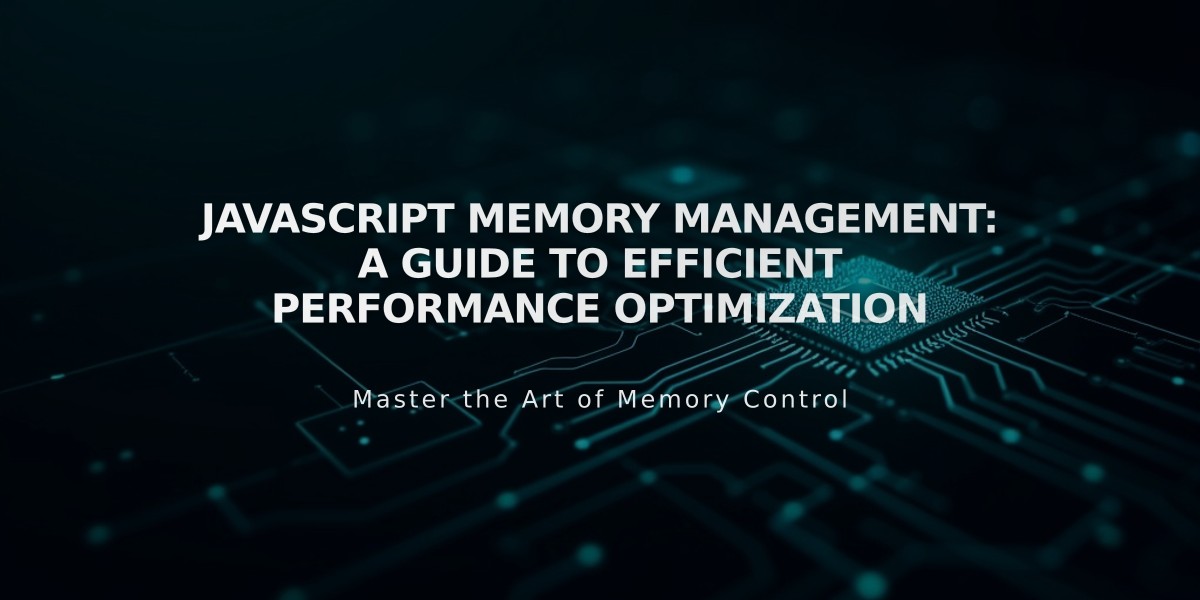
JavaScript Memory Management: A Guide to Efficient Performance Optimization
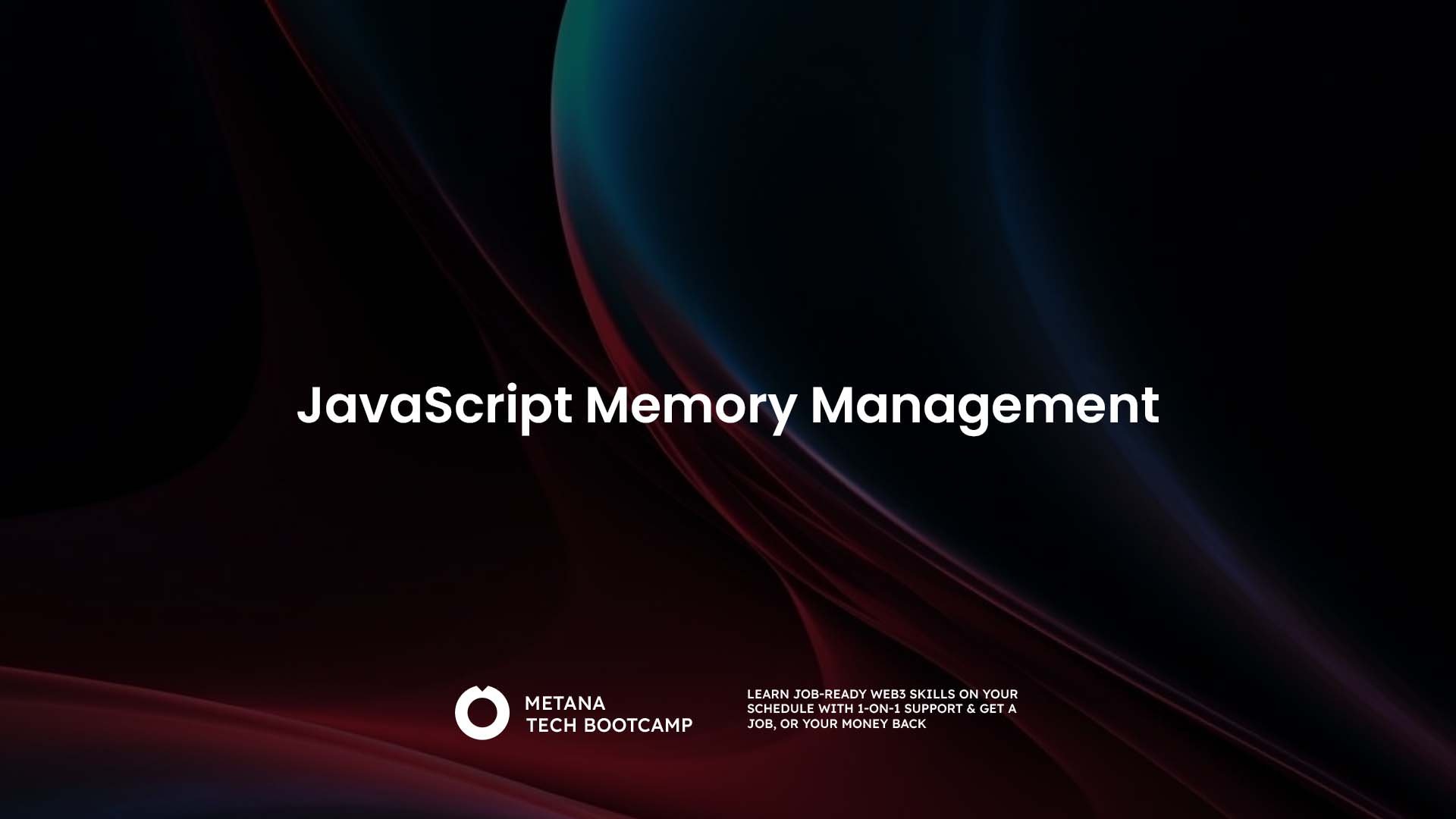
JavaScript Memory Management with dark background
Memory management in JavaScript operates automatically through garbage collection, but understanding its mechanics is crucial for writing efficient applications. The JavaScript engine manages three main memory locations: Stack, Heap, and Queue.
The Stack stores primitive values and function call references, operating on a Last-In-First-Out (LIFO) basis. Each function call creates a new frame containing local variables and references.
The Heap handles complex objects and dynamic memory allocation. This is where objects, arrays, and other reference types are stored. Unlike the Stack, Heap memory isn't organized sequentially.
JavaScript's Garbage Collector (GC) automatically frees memory that's no longer needed. It uses two main algorithms:
- Reference Counting: Tracks how many references point to each object
- Mark and Sweep: Identifies and removes unreachable objects
Common memory leaks occur through:
- Forgotten global variables
- Unclosed event listeners
- Circular references
- Closures retaining large scopes
Best practices for memory management:
- Use local variables instead of global ones
- Remove event listeners when no longer needed
- Clear intervals and timeouts
- Avoid creating unnecessary object references
- Implement proper error handling
- Use WeakMap and WeakSet for temporary references
By monitoring memory usage through Chrome DevTools' Memory panel and implementing these practices, developers can maintain optimal application performance and prevent memory-related issues.
Memory leaks can significantly impact application performance, leading to slower execution and potential crashes. Regular memory profiling and following best practices ensure efficient memory management in JavaScript applications.
Related Articles
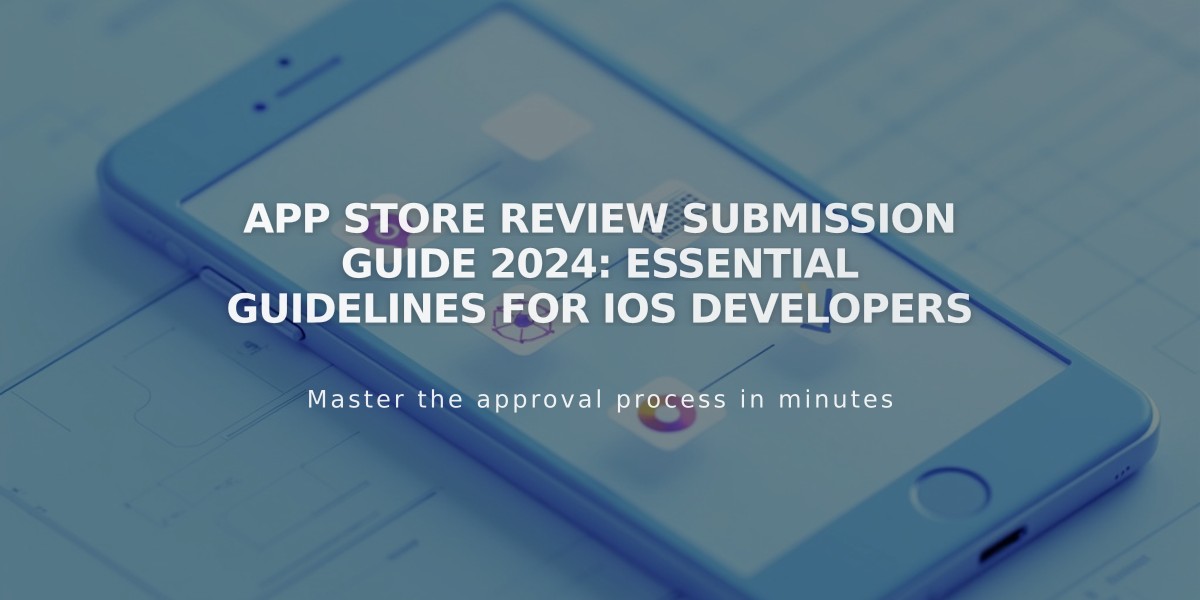
App Store Review Submission Guide 2024: Essential Guidelines for iOS Developers
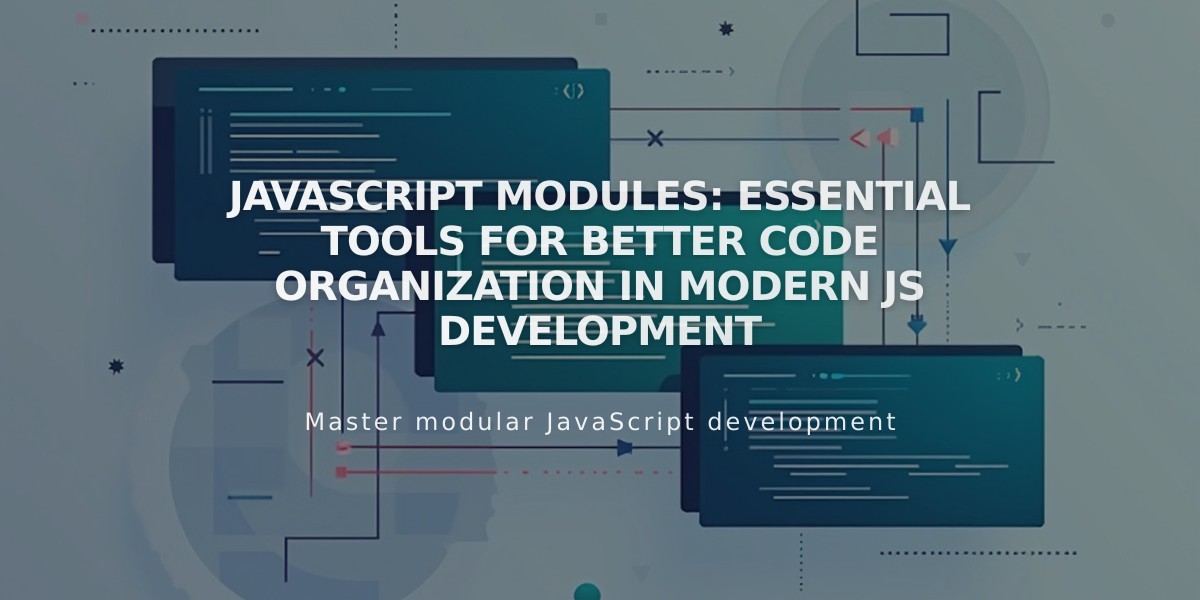