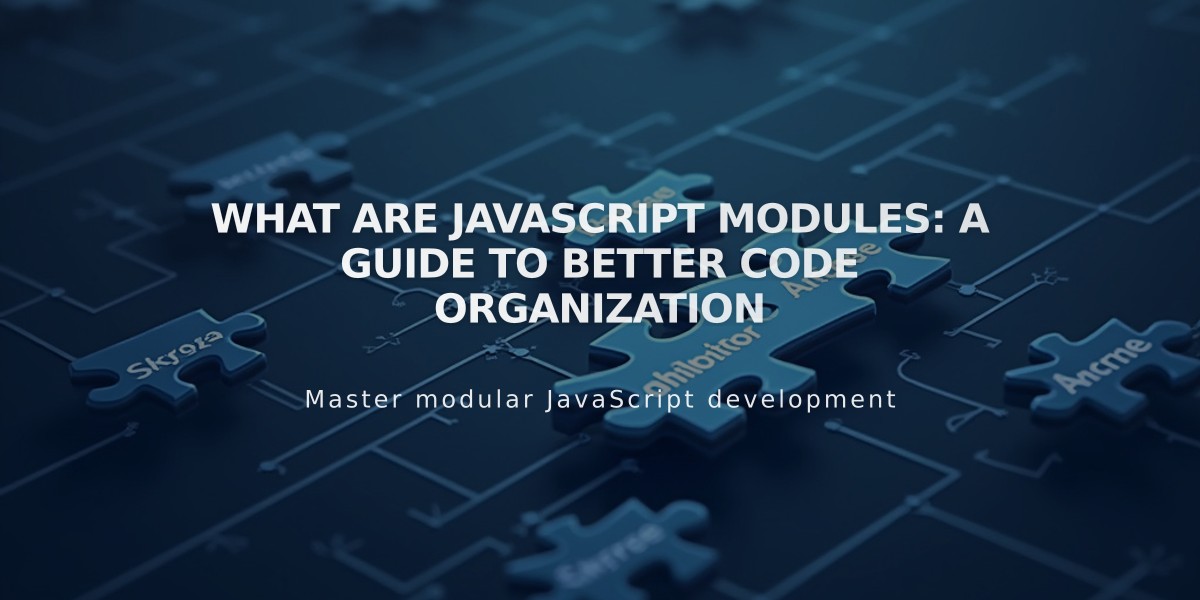
What Are JavaScript Modules: A Guide to Better Code Organization
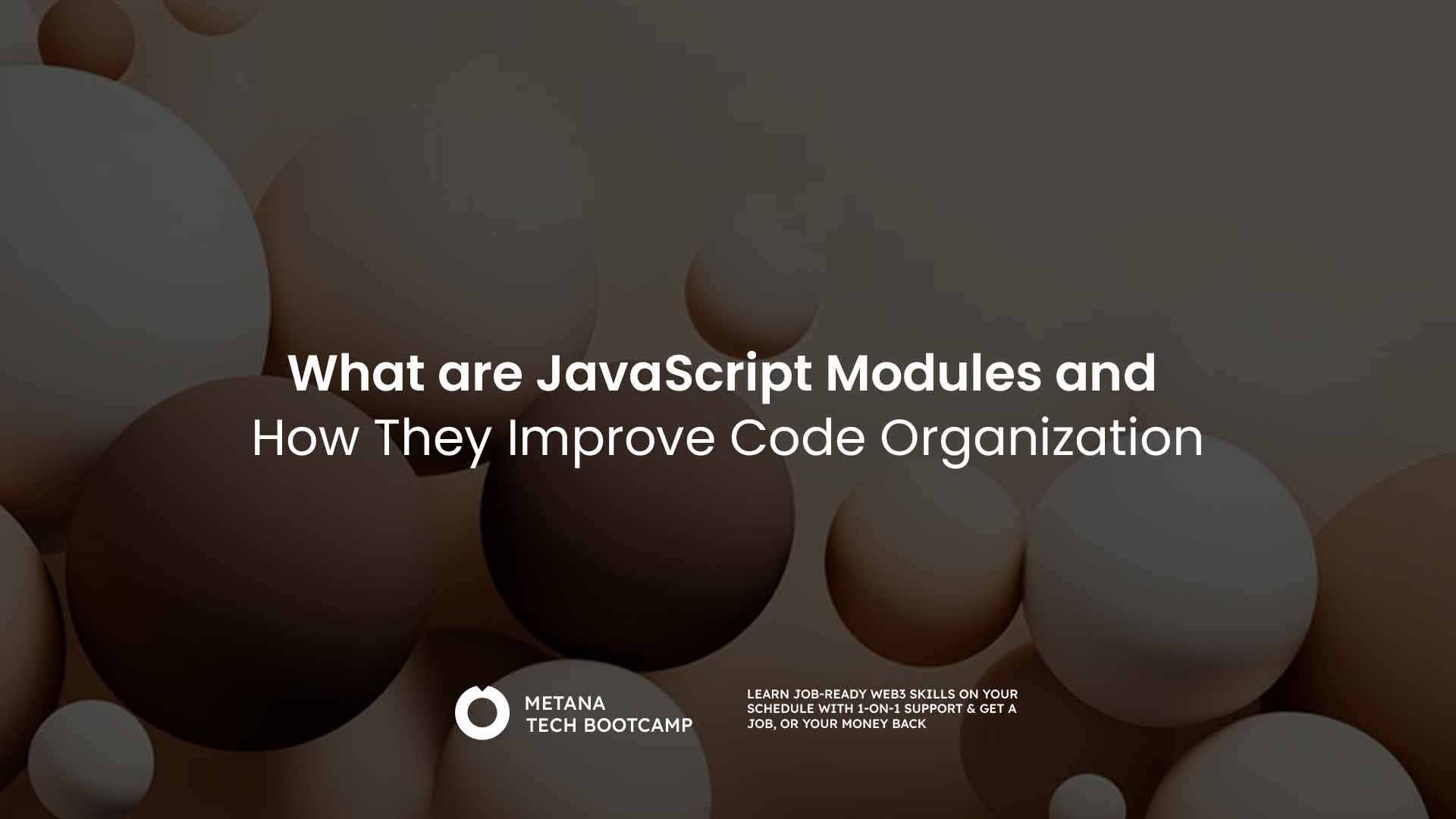
JavaScript modules code structure diagram
JavaScript modules are self-contained units of code that encapsulate related functions, classes, and variables. They provide a structured way to organize code by allowing developers to split functionality into separate files, making applications more maintainable and scalable.
A module can export specific pieces of code that can be imported and used in other modules. This modular approach offers several key benefits:
- Better Code Organization
- Separates code into logical, manageable chunks
- Makes it easier to find and fix bugs
- Improves code readability and maintenance
- Encapsulation
- Keeps private functions and variables hidden
- Reduces naming conflicts
- Prevents unintended modifications to code
- Dependency Management
- Clearly shows relationships between different parts of code
- Makes it easier to track and update dependencies
- Enables better code reusability
Basic Module Syntax:
// exporting from a module export function calculateTotal(items) { return items.reduce((total, item) => total + item.price, 0); } // importing in another file import { calculateTotal } from './calculations.js';
Best Practices for Using Modules:
- Keep modules focused on a single responsibility
- Use descriptive names for modules and their exports
- Maintain clear documentation for module interfaces
- Consider module size and complexity when organizing code
Modules are supported in all modern browsers and can be used with various build tools like Webpack, Rollup, or Parcel. They form the foundation of modern JavaScript applications and are essential for writing clean, maintainable code.
The module system also supports advanced features like:
- Default exports and imports
- Dynamic imports for lazy loading
- Module aggregation
- Circular dependency handling
With proper use of modules, developers can create more robust and scalable applications while maintaining clean and organized codebases.
Related Articles
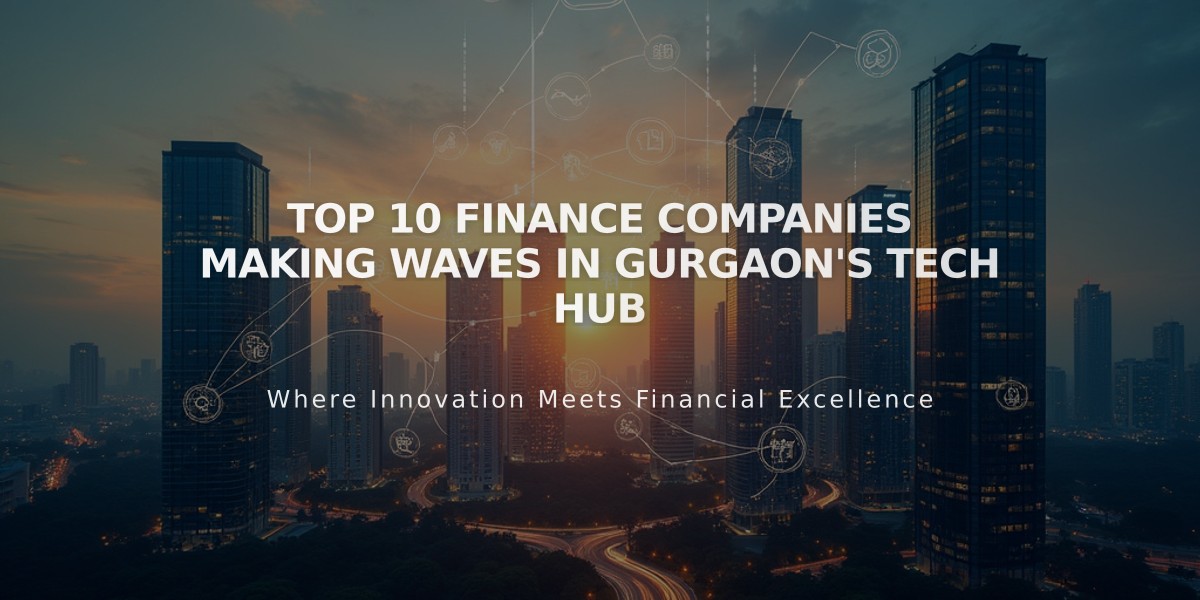
Top 10 Finance Companies Making Waves in Gurgaon's Tech Hub
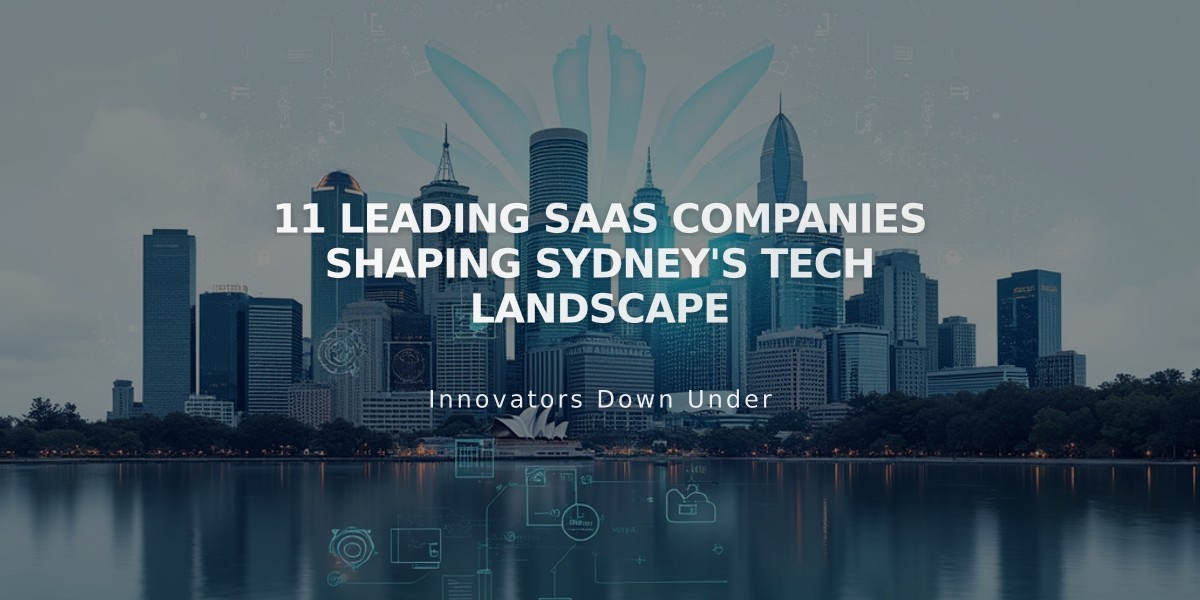